React의 useContext를 활용해도 전역 상태관리가 어느정도는 가능하다.
하지만 프로젝트의 크기가 커질수록 useContext의 부족한 점이 드러나 우리는 라이브러리에서 제공해주는 전역상태관리를 사용하고자 한다.
처음으로 redux를 사용해볼 것이다.
1. redux 설치
yarn add redux react-redux
2. 폴더 및 파일 생성
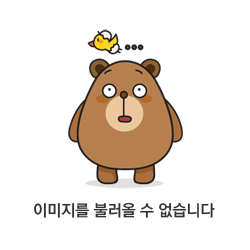
3. configStore 작성
import { combineReducers, createStore } from "redux";
//1) rootReducer 만들기
const rootReducer = combineReducers({});
//2) store 조합
const store = createStore(rootReducer);
//3) store 내보내기
export default store;
4. App컴포넌트 Provider으로 감싸기
import { StrictMode } from "react";
import { createRoot } from "react-dom/client";
import App from "./App.jsx";
import "./index.css";
import { Provider } from "react-redux";
import store from "./redux/config/configStore.jsx";
createRoot(document.getElementById("root")).render(
// <StrictMode>
<Provider store={store}>
<App />
</Provider>
// </StrictMode>
);
이때 Provider의 store은 configStore에서 export한 store이다.
5. 모듈 만들기
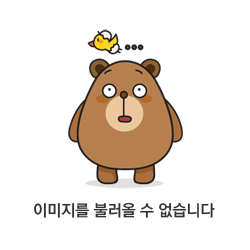
특정 작업을 할 모듈을 만든다.
//초기 상태값
const initialState = {
number: 0,
};
//리듀서(함수)
const counter = (state = initialState, action) => {
switch (action.type) {
default:
return state;
}
};
export default counter;
6. 모듈의 리듀서 store에 연결하기
import { combineReducers, createStore } from "redux";
import counter from "../modules/counter";
//1) rootReducer 만들기
const rootReducer = combineReducers({
counter,
});
//2) store 조합
const store = createStore(rootReducer);
//3) store 내보내기
export default store;
combineReducers에 객체 형식으로 리듀서를 넣어준다.
7. 컴포넌트에서 중앙 저장소(store)의 state값 가져오기
import React from "react";
import { useSelector } from "react-redux";
const App = () => {
const counterReducer = useSelector((state) => {
return state.counter;
});
console.log(counterReducer);
return <div>App</div>;
};
export default App;
useSelector을 사용하여 state의 counter을 가져온다.
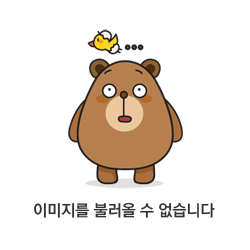
initialState로 지정된 number:0이 저장되어있는 모습이다.
'2차 공부 > TIL' 카테고리의 다른 글
24.08.16 react-router-dom의 부분적 Layout 적용(Outlet) (0) | 2024.08.16 |
---|---|
24.08.16 react-router-Dom 설정 (0) | 2024.08.16 |
24.08.16 리액트 숙련주차 강의 (3) | 2024.08.15 |
24.08.15 챌린지 아티클 -2 / You might not need an effect (0) | 2024.08.15 |
24.08.14 리액트 개념 아티클 (0) | 2024.08.14 |